- 今日推薦開源項目:《綠色回復 DeepCreamPy》
- 今日推薦英文原文:《Recursion — What is it?》
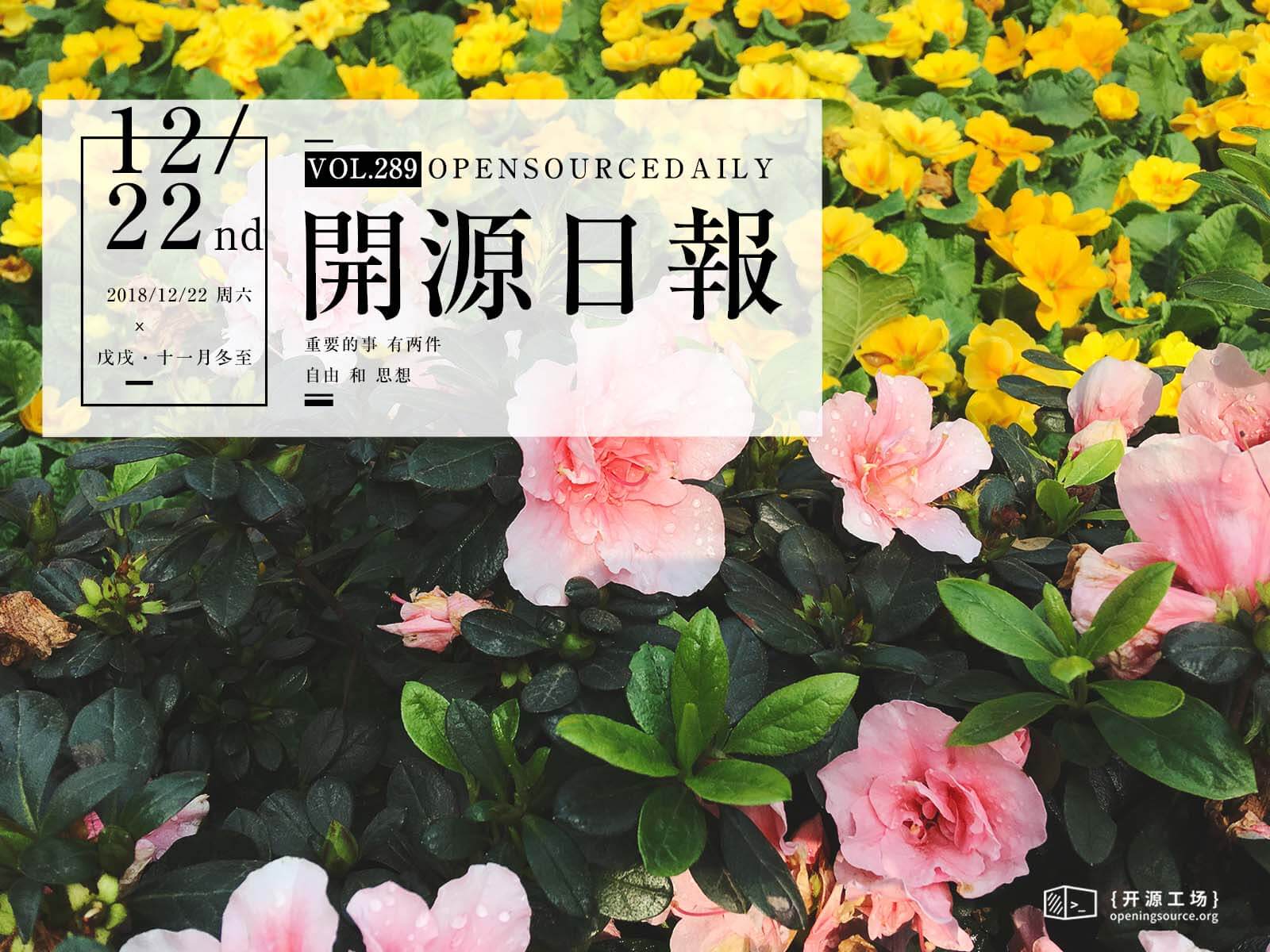
今日推薦開源項目:《綠色回復 DeepCreamPy》
推薦理由:一個基於深度學習的工具,通過神經網路來填充圖片中被刪去的區域(在示例中的綠色區域)。不過實際上,它對其他顏色的抹去也一樣可以進行修復,它最大的局限性在於對現實生活中的照片以及黑白色照片無能為力,而且也沒有辦法對 gif 動圖生效。儘管如此,它還是能對不少照片起到效果,在要用這個工具做什麼上還是請各位自行發揮吧,它能做的可不只是去掉綠色而已。
今日推薦英文原文:《Recursion — What is it?》作者:John Mark Redding
原文鏈接:https://medium.com/@johnmarkredding/recursion-what-is-it-643169503159
推薦理由:這篇文章是面向編程新手的——介紹了遞歸的概念,這玩意是經常用得上的
Recursion — What is it?
We』ll go over the definition of recursion, and some examples of how it could be used. Next we』ll go over the efficiency, and sum up why you might use it. What it is… Recursion is a method of problem solving which relies on recursion to solve the problem. Good definition, right? You』ll see why that sort of makes sense. In the mean-time, here is a better definition: Recursion is a method of problem solving which relies on solving smaller versions of the same problem to complete the solution. In programming, it usually just refers to a function which calls itself. Recursion: a function which calls itself Sounds simple, right, but this can get you in trouble.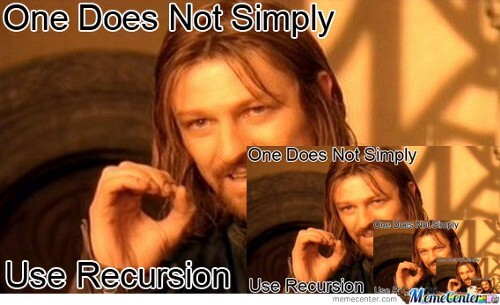
def factorial(num)
#base case – the point at which the problem stops calling itself.
if num == 2
2
else
num * factorial(num - 1)
end
end
puts factorial(gets.chomp.to_i)
factorial(4) => 24
In math, this would essentially be: 4 × 3 × 2 = 24.
We don』t multiply by 1 as the result will be the same.
This looks rather elegant. Get a number, if it is 2, return it, otherwise, multiply, and break it down.
Palindrome.js
function pal(word) {
if (word.length <= 1) {
return true
} else {
let fChar = word.shift()
let lChar = word.pop()
if (fChar == lChar) {
return pal(word)
} else {
return false
}
}
}
let eve = ['e','v','e']
let steve = ['s','t','e','v','e']
console.log(`Is ${eve.join("")} a palindrome: ${pal(eve)}`)
console.log(`Is ${steve.join("")} a palindrome: ${pal(steve)}`)
This is essentially what the function is checking, though you may not have the reverse function available to you in all projects.
"Steve".reverse() == "Steve" => false
"eve".reverse() == "eve" => true
Efficiency
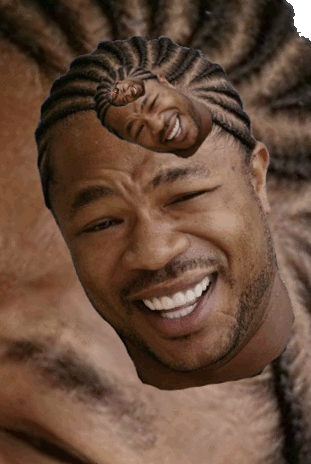
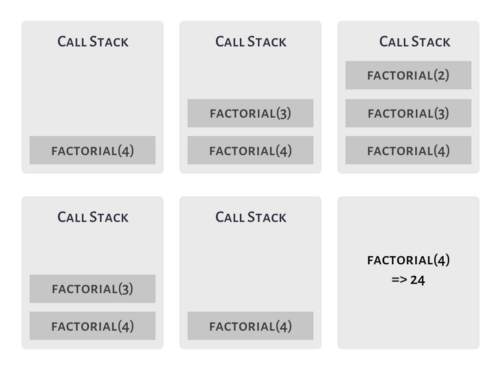