今日推荐开源项目:《基础表情图标 twemoji》
今日推荐英文原文:《Stop Putting So Many If Statements in Your JavaScript》
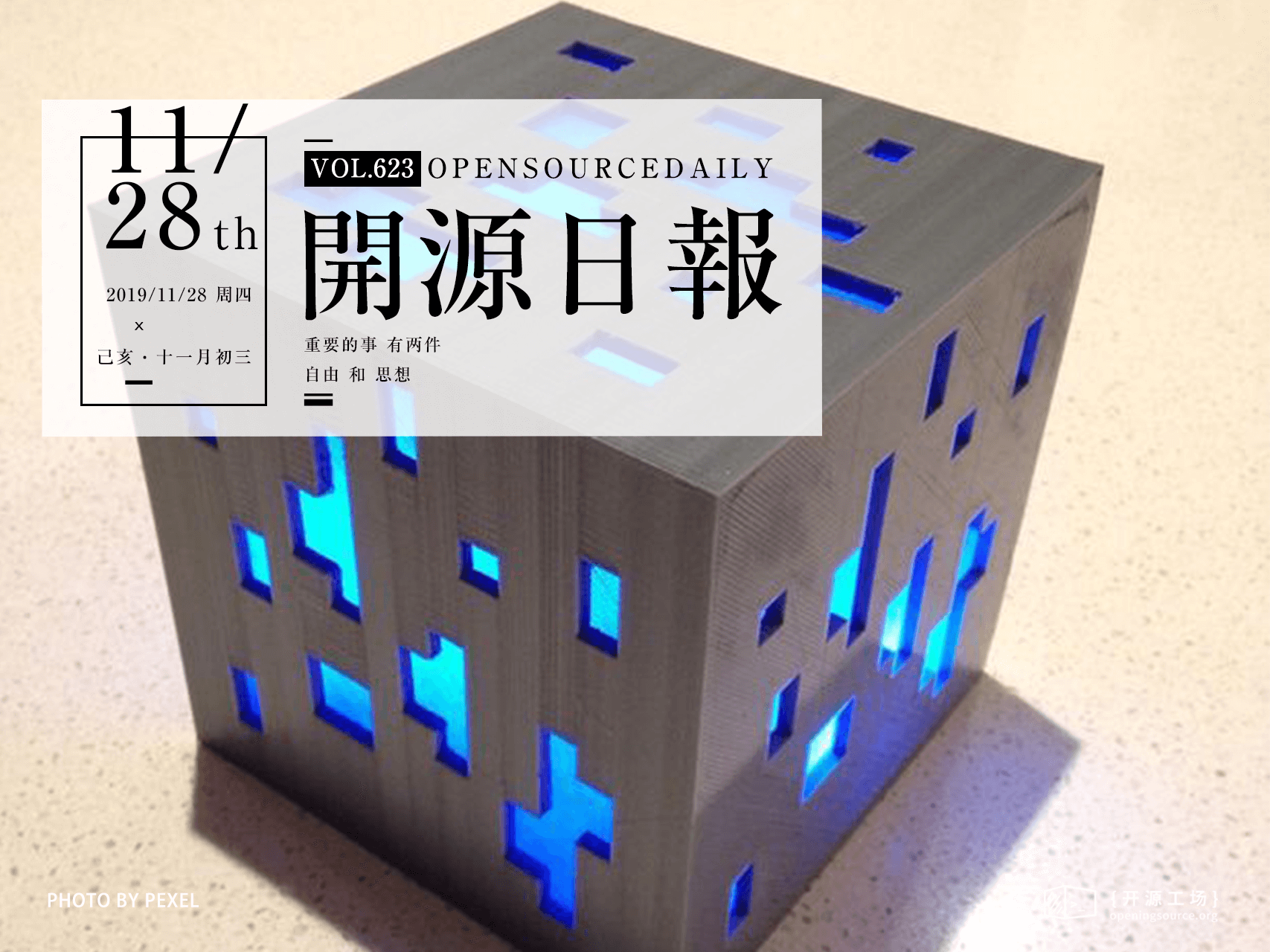
今日推荐开源项目:《基础表情图标 twemoji》传送门:GitHub链接
推荐理由:就如同那些叫做 xxxIcon 的图标库一样,表情图标一样可以装点页面,而且其拥有各种颜色的特点比起大部分时候都是纯色的普通图标来说更适合于需要花哨装扮的页面。这个项目是推特的基础 Unicode 表情符号库,可以通过使用 API 或者直接插入 PNG、SVG 等方式来加入页面,在使用前别忘了加上 UTF-8 的字符集限定。
今日推荐英文原文:《Stop Putting So Many If Statements in Your JavaScript》作者:Chris Geelhoed
原文链接:https://medium.com/better-programming/stop-putting-so-many-if-statements-in-your-javascript-3b65aaa4b86b
推荐理由:if 的另外四种写法,有些时候能够保持易读性的同时让代码更简洁
Stop Putting So Many If Statements in Your JavaScript
4 other ways of handling conditional logic in your code
“To a man with a hammer, everything looks like a nail” — Mark TwainI like to think of conditional logic as the bread and butter of software. It gives developers the power to build things that are interesting, useful, and fun.
The most popular way of handling conditional logic is the if statement. The if statement is universal, flexible, and easy to understand, so its popularity comes as no surprise.
There are, however, other ways of handling conditional logic that are often overlooked by developers. It’s easy to reach for the trusty if statement for every job, but learning about other techniques will make you a more skillful and efficient programmer.
A master carpenter wouldn’t use the same tool for every job, and a master developer shouldn’t either.
This article explores four alternatives to the classic if statement.
- Ternary Operators
- Switch Statements
- Logical Operators (&& and ||)
- Lookup Maps
Ternary Operators
Ternary operators are a great way to handle basic if-else conditions. In the example below the value assigned to message changes depending on whether hasError is truthy or falsy.With a classic if statement:
let message = "Thanks for contacting us! We'll be in touch shortly!"
if (hasError) {
message = "Oops, that's an error. Please try again later!"
}
This solution first sets the message to be the error-free version and then overwrites it if necessary.Now with a ternary operator instead:
const message = hasError
? "Oops, that's an error. Please try again later!"
: "Thanks for contacting us! We'll be in touch!"
The ternary option has some notable advantages here:
- It’s more compact because message only needs to be assigned once.
- Since the message no longer needs to be overwritten when an error is present, we can use const instead of let.
Switch Statements
Switch statements are the most obvious substitute for if statements. Instead of determining whether a condition is truthy or falsy, it looks at one specific value and executes it’s matching case block.This makes switch statements a little less flexible than if statements, but it also makes them a little more concise. Let’s look at an example:
First, with an if statement:
if (status === 200) {
handleSuccess()
} else if (status === 401) {
handleUnauthorized()
} else if (status === 404) {
handleNotFound()
} else {
handleUnknownError()
}
Next, with a switch:
switch (status) {
case 200:
handleSuccess()
break
case 401:
handleUnauthorized()
break
case 404:
handleNotFound()
break
default:
handleUnknownError()
break
}
The switch uses more lines of code, but avoids repeating the equality check again and again, and has a more streamlined appearance overall.One important detail to consider when writing switch statements is your use of breaks. Unlike an if-else chain, switch statements can “fall through” to the next case. This can be confusing, so it’s usually recommended that you add a break to the end of every case.
Logical Operators (&& and ||)
The && and || (“and” and “or”) operators behave differently in JavaScript then they do in other programming languages. This special behavior gives these operators a unique ability to handle conditional logic.Here’s how the && operator works in JavaScript:
- First, it looks at the left side of the operator. If its value is falsy then it returns that value without even looking at the right side of the operator.
- If the value on the left side is found to be truthy then the value on the right of the operator is returned.
- First, it looks at the left side of the operator. If its value is truthy then it returns that value without even looking at the right side of the operator.
- If the value on the left side is found to be falsy then the value on the right of the operator is returned.
Putting the && Operator into Action
Often you want to access a property inside an object, but can’t be sure whether that object exists beforehand.For example, maybe you want to use a user’s name property to construct a welcome message:
const message = `Welcome, ${user.name}!`
But what if user is set to null, false, or undefined instead?
const message = `Welcome, ${user.name}!`
// TypeError: Cannot read property 'name' of null
If user is not an object and we attempt to access the name property on it, JavaScript will throw an error.This can be avoided by adding an if statement to your code:
let message = null
if (user && user.name) {
message = `Welcome, ${user.name}!`
}
This does the trick, but && operators can make this a bit more concise:
const message = user && user.name && `Welcome, ${user.name}!`
This approach allows for message to be set with const rather than let and gets the job done in a single line of code. Much better!
Using the || Operator
The || operator is great for assigning fallback values.As an example, let’s say that you want to create a handle variable for your current user. If that user has a valid username then that should be used, but if the username is set to null then a fallback value of “Guest” should be used instead.
First, with a plain if statement:
let handle = 'Guest'
if (username) {
handle = username
}
Now, by using the || operator:
const handle = username || 'Guest'
Again — much cleaner and only one line of code. A great improvement!
Lookup Maps
Lookup maps are perfect for getting one value that’s associated with another.As an example, imagine that we want to get the color associated with the status of a message box. A typical setup might look something like this:
- Success is green
- Warning is yellow
- Info is blue
- Error is red
function getStatusColor (status) {
if (status === 'success') {
return 'green'
}
if (status === 'warning') {
return 'yellow'
}
if (status === 'info') {
return 'blue'
}
if (status === 'error') {
return 'red'
}
}
This is fine, but a lookup map might be more suitable. Object literals are one way to implement a lookup map in JavaScript:
function getStatusColor (status) {
return {
success: 'green',
warning: 'yellow',
info: 'blue',
error: 'red'
}[status]
}
This is slimmer and less repetitive. As an added benefit, lookup maps don’t necessarily need to be hardcoded — the relationship between status’ and colors could be dynamic and this pattern would still work.
Summary
If statements are a powerful tool that all JavaScript developers should keep close at hand, but there are other methods of handling conditional logic that are sometimes more appropriate.Ternary operators are perfect for handling if-else logic in a single line of code, but they should only be used for fairly simple use cases.
Switch statements are ideal when you are interested in a specific variable that could take on multiple distinct values. They are less powerful than if statements but are often nicer to look at.
Unlike other programming languages, the && and || operators don’t always return a boolean value in JavaScript, and this behavior can be useful. The && operator is often used to avoid errors when attempting to access an object’s properties, and the || operator is often used to assign a fallback value to a variable when a first choice is not available.
Lookup maps are a great way to get one value that is associated with another, for example getting the color associated with a message’s status (a status of success might map to a color of green).
Mastering these four conditional logic patterns will give you more flexibility in how you structure your JavaScript and will make you a better programmer overall. By selecting the right tool for the job, your code will become more elegant, concise, and maintainable.
下载开源日报APP:https://openingsource.org/2579/
加入我们:https://openingsource.org/about/join/
关注我们:https://openingsource.org/about/love/