今日推荐开源项目:《即刻 CopyTranslator》
今日推荐英文原文:《Stop Using Else in Your Programs》
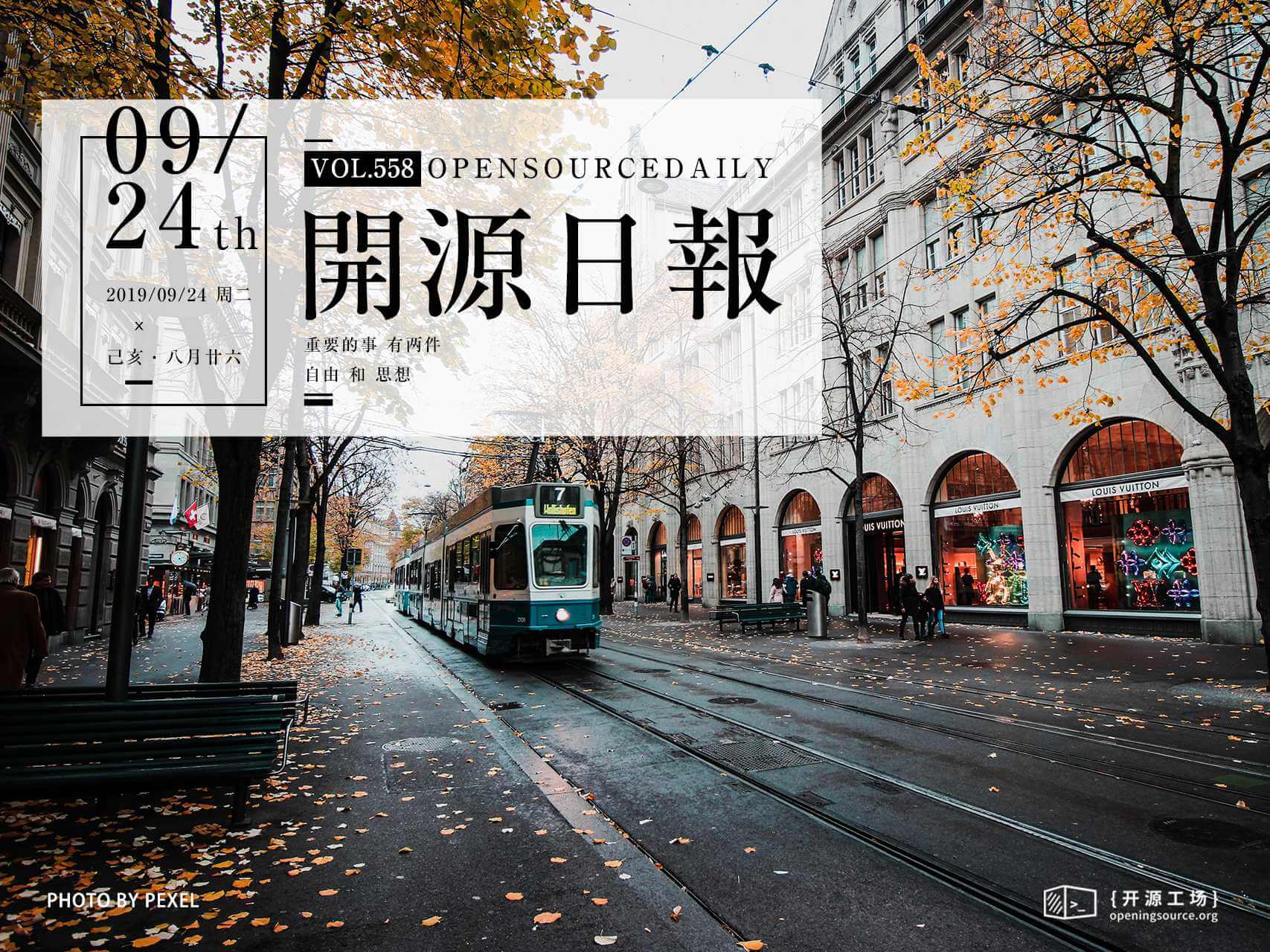
今日推荐开源项目:《即刻 CopyTranslator》传送门:GitHub链接
推荐理由:读英文文档的时候基本上都会需要一个翻译器来辅助——即使读得多了也没准就有啥词给突然忘了呢。这个项目可以读取你的剪贴板,当你复制一段想要翻译的文字之后就会响应将其自动翻译,不需要额外的粘贴到其他翻译器上,也克服了划词翻译对大段文字的不适应,作为提升工作效率的道具来说相当合适,也能够在平时作为单纯的划词翻译来练习阅读能力。
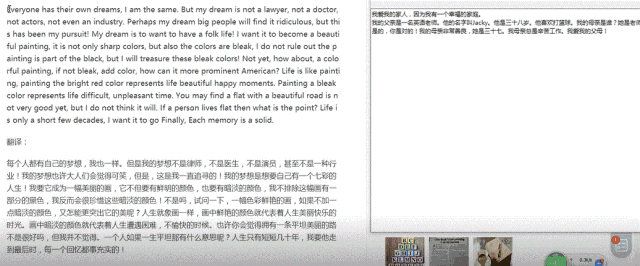
今日推荐英文原文:《Stop Using Else in Your Programs》作者:Joey Colon
原文链接:https://medium.com/better-programming/why-you-need-to-stop-using-else-statements-5b1fd09dea9e
推荐理由:在多个条件判定时一种比起堆叠 if-else 来说更整洁的写法
Stop Using Else in Your Programs
A practical introduction to guard clauses
When I first began programming, I wish someone would have told me about a few different concepts to think about when writing my first website.One of the mistakes I fell into when starting was overusing the else keyword when writing conditionals. This is something that I find plenty of others doing in their own code as well, so I thought I should shed some light on the topic.
A disclaimer; my view on this topic is purely subjective.
In some situations, you may not be able to apply this type of approach to your code. Sometimes, the solution is to use the else keyword. This post is to inform people of an alternative approach to structuring logic.
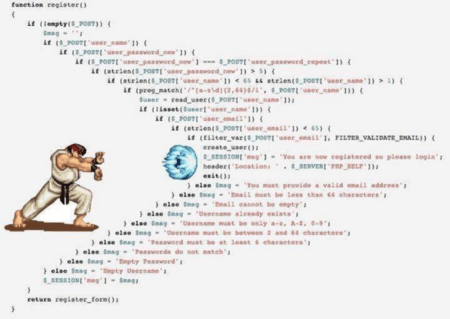
Indent Hadouken
Guard Clauses
According to Wikipedia, a guard clause is a check of integrity preconditions used to avoid errors during execution.Let’s break down that definition into layman’s terms. We perform a check of integrity preconditions (at the beginning of our code) to avoid any bugs during the main flow of our logic.
In the perfect flow (when validation is correct), we want the main logic of our program to be after all of the validation checks.
Let’s imagine that we run a website where we have a premium purchase area that is restricted to paying customers only and only open after 12 pm.
<?php
if ($user != null) {
if (time() >= strtotime('12 pm')) {
if ($user->hasAccess(UserType.PREMIUM)) {
if ($store->hasItemsInStock()) {
// the content a premium user user should be able to see when the store is in stock
// after 12pm.
} else {
return 'We are completely sold out.';
}
} else {
return 'You do not have premium access to our website.';
}
} else {
return 'This section is not opened before 12PM';
}
} else {
return 'You are not signed in.';
}
In an actual application, we would likely return some form of exception.While this is an approach to flow through the conditionals, the else keywords become difficult to follow even when we only have a small number of them.
This is a trivial example of conditional logic but, out in the wild, imagine trying to navigate a class that has much more complex logic. Coding through this standard, in my opinion, is not sustainable and we should do better.
With guard clauses, we will want to follow this framework:
<?php
if (condition1()) {
return ...;
}
if (condition2()) {
return ...;
}
// Input is valid.
doSomething();
Taking that framework, we can restructure the previous code like the following:
<?php
if ($user == null) {
return 'You are not signed in.';
}
if (time() < strtotime('12 pm')) {
return 'This section is not opened before 12PM';
}
if (!$user->hasAccess(UserType.PREMIUM)) {
return 'You do not have premium access to our website';
}
if (!$store->hasItemsInStock()) {
return 'We are completely sold out.';
}
// the content a premium user user should be able to see when the store is in stock
// after 12pm.
In guard clauses, we typically invert the boolean expression to what we want to assert. If we want the user to be signed in to view this page, we want to check if they’re not signed in.This approach captures the same exact logic flow but, in my opinion and in others as well, this is a much cleaner approach when tackling conditional logic.
Conclusion
When coding, we should always keep the question in-mind of: “How easy will this be to maintain 6 months down the line?”Creating solutions to a problem at the current time is great. But what about in the future? Not building software with the future in-mind is silly.
It has personally caused me to entirely rewrite whole features from scratch because of how much technical debt I accumulated over time through numerous band-aid fixes.
Utilizing guard clauses will set your future self/team up for success for when new requirements are added to your program.
下载开源日报APP:https://openingsource.org/2579/
加入我们:https://openingsource.org/about/join/
关注我们:https://openingsource.org/about/love/